Strands Agents
Strands Agents is a simple yet powerful SDK that takes a model-driven approach to building and running AI agents. From simple conversational assistants to complex autonomous workflows, from local development to production deployment, Strands Agents scales with your needs.
Opik’s integration with Strands Agents relies on OpenTelemetry. You can learn more about Opik’s OpenTelemetry features in our get started guide.
Getting started
To use the Strands Agents integration with Opik, you will need to have the following packages installed:
In addition, you will need to set the following environment variables to configure the OpenTelemetry integration:
Opik Cloud
Self-hosted instance
If you are using Opik Cloud, you will need to set the following environment variables:
To log the traces to a specific project, you can add the
projectName
parameter to the OTEL_EXPORTER_OTLP_HEADERS
environment variable:
You can also update the Comet-Workspace
parameter to a different
value if you would like to log the data to a different workspace.
Using Opik with Strands Agents
The example below shows how to use the Strands Agents integration with Opik:
Once the integration is set-up, you will see the trace in Opik:
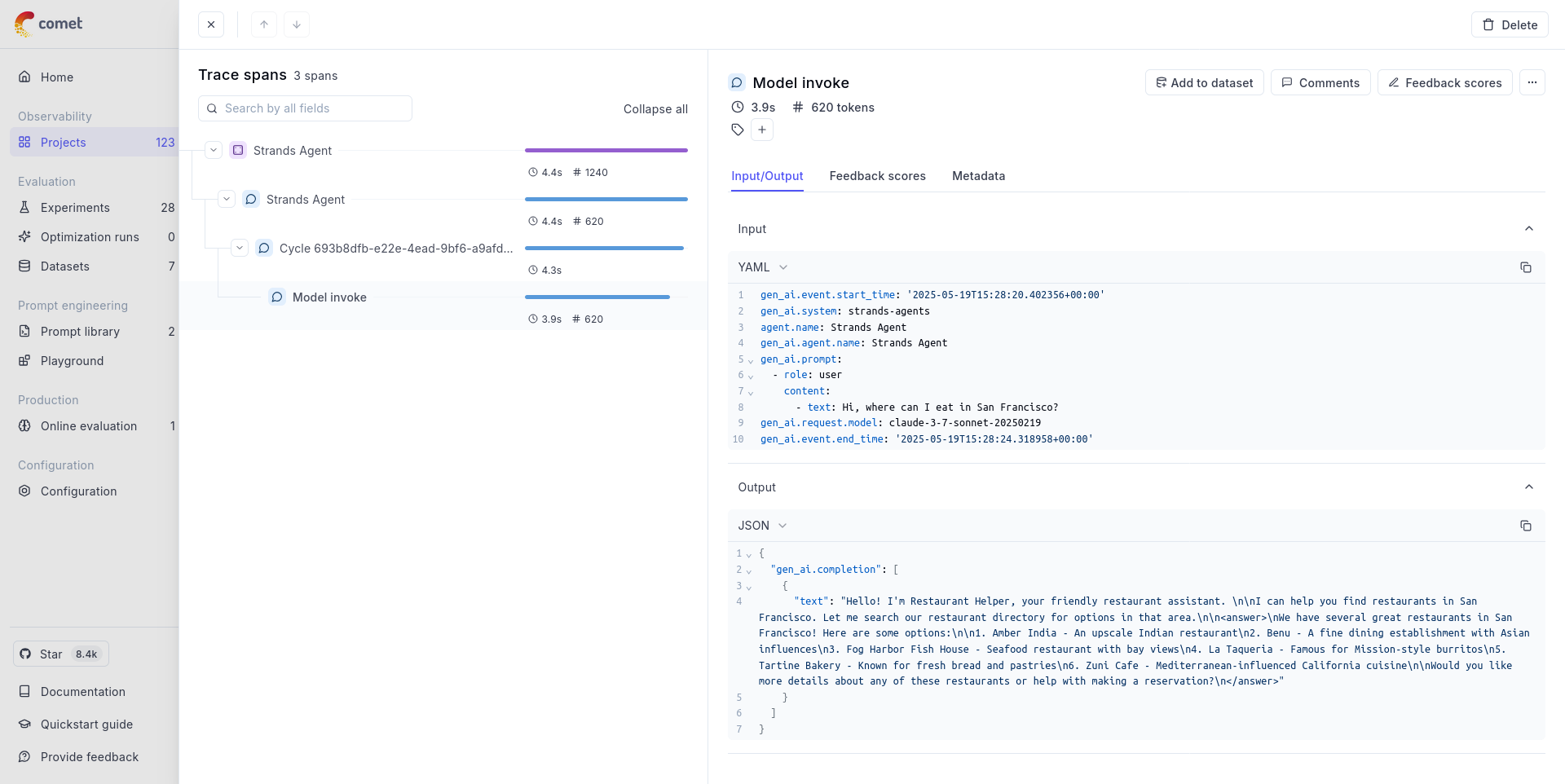