Agent Development Kit
Agent Development Kit (ADK) is a flexible and modular framework for developing and deploying AI agents. ADK can be used with popular LLMs and open-source generative AI tools and is designed with a focus on tight integration with the Google ecosystem and Gemini models. ADK makes it easy to get started with simple agents powered by Gemini models and Google AI tools while providing the control and structure needed for more complex agent architectures and orchestration.
Opik integrates with ADK to log traces for all ADK agent execution.
Getting started
First, ensure you have both opik
and google-adk
installed:
In addition, you can configure Opik using the opik configure
command which will prompt you for the correct local server address or if you are using the Cloud platform your API key:
Logging ADK agent executions
To log a ADK agent run, you can use the OpikTracer
. This connector will log the agent run to the Opik platform:
Each agent execution will now be logged to the Opik platform:
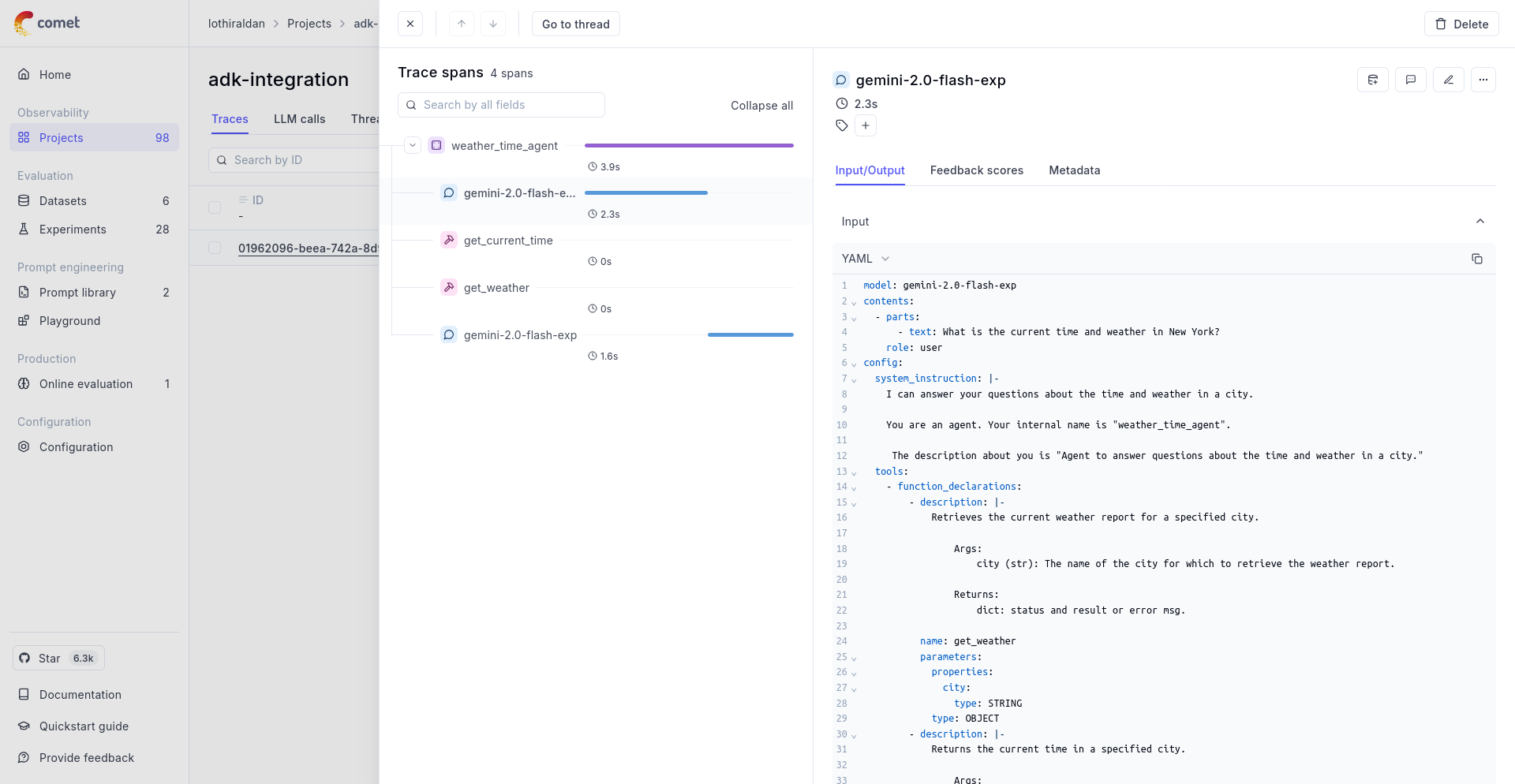