Opik Agent Optimizer: Getting Started
This guide will help you get started with Opik Agent Optimizer SDK for improving your LLM prompts through systematic optimization.
Prerequisites
- Python 3.9 or higher
- An Opik API key (sign up here if you don’t have one)
Getting Started with Optimizers
Here’s a step-by-step guide to get you up and running with Opik Agent Optimizer:
1. Install Opik and the optimizer package
Install the required packages using pip or uv (recommended for faster installation):
Then configure your Opik environment:
2. Import necessary modules
First, we import all the required classes and functions from opik
and opik_optimizer
.
3. Define your evaluation dataset
You can use a demo dataset for testing, or create/load your own. You can learn more about creating datasets in the Manage Datasets documentation.
4. Configure the evaluation metric
metric
tells the optimizer how to score the LLM’s outputs. Here, LevenshteinRatio
measures the similarity between the model’s response and a “label” field in the dataset.
5. Define your base prompt
This is the initial instruction that the MetaPromptOptimizer
will try to enhance:
6. Choose and configure an optimizer
Instantiate MetaPromptOptimizer
, specifying the model to be used in the optimization
process.
7. Run the optimization
The optimizer.optimize_prompt(...)
method is called with the dataset, metric configuration,
and prompt to start the optimization process.
9. View results in the CLI
After optimization completes, call result.display()
to see a summary of the optimization,
including the best prompt found and its score, directly in your terminal.
The OptimizationResult
object also contains more details in result.history
and
result.details
. The optimization results will be displayed in your console, showing the
progress and final scores.
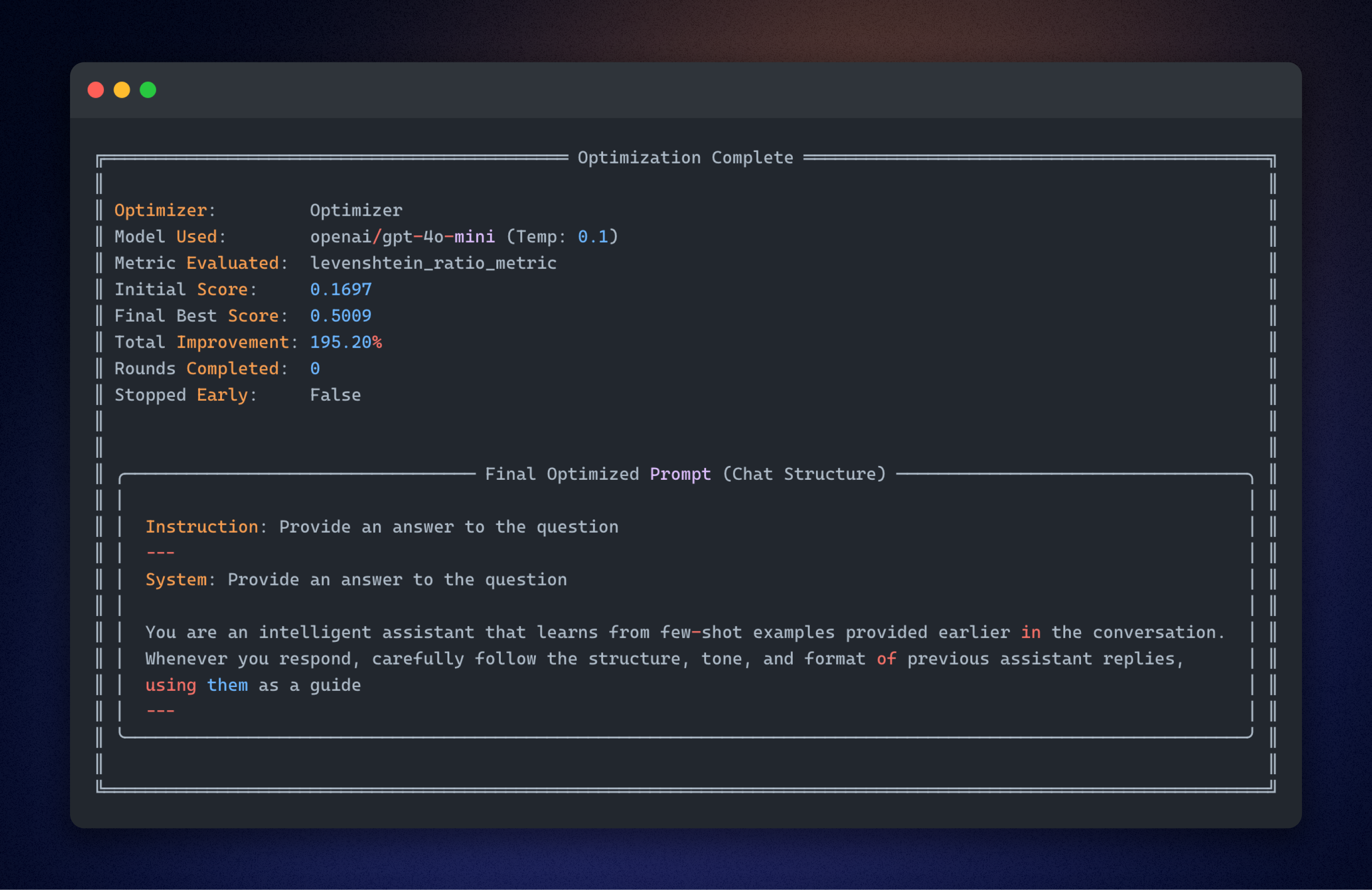
Next Steps
- Explore different optimization algorithms to choose the best one for your use case
- Understand prompt engineering best practices
- Set up your own evaluation datasets
- Review the API reference for detailed configuration options
🚀 Want to see Opik Agent Optimizer in action? Check out our Example Projects & Cookbooks for runnable Colab notebooks covering real-world optimization workflows, including HotPotQA and synthetic data generation.